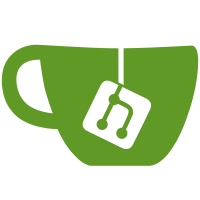
A previous fix for SPIGOT-6814 implemented a callback function for the PDC implementation that could be set to actively define a chunk as unsaved, allowing chunks that have not been mutated through block changes to still require saving if the chunks pdc was mutated. This implementation however would pass a callback that references the chunk access internally, meaning the PDC now actively holds onto a callback that holds a reference to the entire chunk. Aditionally, this change also impacted the pdc for item metas and entities for really no reason whatsoever. This commit re-implements the fix by introducing a new child of the pdc implementation that the chunk now uses as its pdc. This specific implementation maintains a dirty flag that is set to `true` on any form of mutation and set back to false by the chunk that owns the PDC whenever the chunk itself is flag as no longer dirty.
88 lines
3.8 KiB
Diff
88 lines
3.8 KiB
Diff
--- a/net/minecraft/world/level/chunk/IChunkAccess.java
|
|
+++ b/net/minecraft/world/level/chunk/IChunkAccess.java
|
|
@@ -77,6 +77,11 @@
|
|
protected final LevelHeightAccessor levelHeightAccessor;
|
|
protected final ChunkSection[] sections;
|
|
|
|
+ // CraftBukkit start - SPIGOT-6814: move to IChunkAccess to account for 1.17 to 1.18 chunk upgrading.
|
|
+ private static final org.bukkit.craftbukkit.persistence.CraftPersistentDataTypeRegistry DATA_TYPE_REGISTRY = new org.bukkit.craftbukkit.persistence.CraftPersistentDataTypeRegistry();
|
|
+ public org.bukkit.craftbukkit.persistence.DirtyCraftPersistentDataContainer persistentDataContainer = new org.bukkit.craftbukkit.persistence.DirtyCraftPersistentDataContainer(DATA_TYPE_REGISTRY);
|
|
+ // CraftBukkit end
|
|
+
|
|
public IChunkAccess(ChunkCoordIntPair chunkcoordintpair, ChunkConverter chunkconverter, LevelHeightAccessor levelheightaccessor, IRegistry<BiomeBase> iregistry, long i, @Nullable ChunkSection[] achunksection, @Nullable BlendingData blendingdata) {
|
|
this.chunkPos = chunkcoordintpair;
|
|
this.upgradeData = chunkconverter;
|
|
@@ -94,7 +99,11 @@
|
|
}
|
|
|
|
replaceMissingSections(levelheightaccessor, iregistry, this.sections);
|
|
+ // CraftBukkit start
|
|
+ this.biomeRegistry = iregistry;
|
|
}
|
|
+ public final IRegistry<BiomeBase> biomeRegistry;
|
|
+ // CraftBukkit end
|
|
|
|
private static void replaceMissingSections(LevelHeightAccessor levelheightaccessor, IRegistry<BiomeBase> iregistry, ChunkSection[] achunksection) {
|
|
for (int i = 0; i < achunksection.length; ++i) {
|
|
@@ -258,10 +267,11 @@
|
|
|
|
public void setUnsaved(boolean flag) {
|
|
this.unsaved = flag;
|
|
+ if (!flag) this.persistentDataContainer.dirty(false); // CraftBukkit - SPIGOT-6814: chunk was saved, pdc is no longer dirty
|
|
}
|
|
|
|
public boolean isUnsaved() {
|
|
- return this.unsaved;
|
|
+ return this.unsaved || this.persistentDataContainer.dirty(); // CraftBukkit - SPIGOT-6814: chunk is unsaved if pdc was mutated
|
|
}
|
|
|
|
public abstract ChunkStatus getStatus();
|
|
@@ -394,6 +404,27 @@
|
|
}
|
|
}
|
|
|
|
+ // CraftBukkit start
|
|
+ public void setBiome(int i, int j, int k, BiomeBase biome) {
|
|
+ try {
|
|
+ int l = QuartPos.fromBlock(this.getMinBuildHeight());
|
|
+ int i1 = l + QuartPos.fromBlock(this.getHeight()) - 1;
|
|
+ int j1 = MathHelper.clamp(j, l, i1);
|
|
+ int k1 = this.getSectionIndex(QuartPos.toBlock(j1));
|
|
+
|
|
+ this.sections[k1].setBiome(i & 3, j1 & 3, k & 3, biome);
|
|
+ } catch (Throwable throwable) {
|
|
+ CrashReport crashreport = CrashReport.forThrowable(throwable, "Setting biome");
|
|
+ CrashReportSystemDetails crashreportsystemdetails = crashreport.addCategory("Biome being set");
|
|
+
|
|
+ crashreportsystemdetails.setDetail("Location", () -> {
|
|
+ return CrashReportSystemDetails.formatLocation(this, i, j, k);
|
|
+ });
|
|
+ throw new ReportedException(crashreport);
|
|
+ }
|
|
+ }
|
|
+ // CraftBukkit end
|
|
+
|
|
public void fillBiomesFromNoise(BiomeResolver biomeresolver, Climate.Sampler climate_sampler) {
|
|
ChunkCoordIntPair chunkcoordintpair = this.getPos();
|
|
int i = QuartPos.fromBlock(chunkcoordintpair.getMinBlockX());
|
|
@@ -425,8 +456,10 @@
|
|
return this;
|
|
}
|
|
|
|
- public static record a(SerializableTickContainer<Block> a, SerializableTickContainer<FluidType> b) {
|
|
+ // CraftBukkit start - decompile error
|
|
+ public static record a(SerializableTickContainer<Block> blocks, SerializableTickContainer<FluidType> fluids) {
|
|
|
|
+ /*
|
|
private final SerializableTickContainer<Block> blocks;
|
|
private final SerializableTickContainer<FluidType> fluids;
|
|
|
|
@@ -442,5 +475,7 @@
|
|
public SerializableTickContainer<FluidType> fluids() {
|
|
return this.fluids;
|
|
}
|
|
+ */
|
|
+ // CraftBukkit end
|
|
}
|
|
}
|