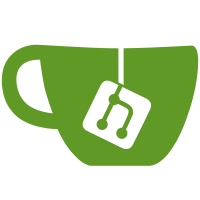
- SPIGOT-5732: Fix issue with spawning leash hitches and painting, by using the right block faces - SPIGOT-6387: Allow hanging entities to be spawned mid air - Use randomize parameter to determine if a random painting should be chosen or not - Return BlockFace self by leash hitches entity - Throw a standardised exception when trying to set a BlockFace to a hanging entity which the entity does not support, instead of using BlockFace south or throwing a null pointer
76 lines
2.2 KiB
Java
76 lines
2.2 KiB
Java
package org.bukkit.craftbukkit.entity;
|
|
|
|
import net.minecraft.core.EnumDirection;
|
|
import net.minecraft.world.entity.decoration.EntityHanging;
|
|
import org.bukkit.block.BlockFace;
|
|
import org.bukkit.craftbukkit.CraftServer;
|
|
import org.bukkit.craftbukkit.block.CraftBlock;
|
|
import org.bukkit.entity.EntityType;
|
|
import org.bukkit.entity.Hanging;
|
|
|
|
public class CraftHanging extends CraftEntity implements Hanging {
|
|
public CraftHanging(CraftServer server, EntityHanging entity) {
|
|
super(server, entity);
|
|
}
|
|
|
|
@Override
|
|
public BlockFace getAttachedFace() {
|
|
return getFacing().getOppositeFace();
|
|
}
|
|
|
|
@Override
|
|
public void setFacingDirection(BlockFace face) {
|
|
setFacingDirection(face, false);
|
|
}
|
|
|
|
@Override
|
|
public boolean setFacingDirection(BlockFace face, boolean force) {
|
|
EntityHanging hanging = getHandle();
|
|
EnumDirection dir = hanging.getDirection();
|
|
switch (face) {
|
|
case SOUTH:
|
|
getHandle().setDirection(EnumDirection.SOUTH);
|
|
break;
|
|
case WEST:
|
|
getHandle().setDirection(EnumDirection.WEST);
|
|
break;
|
|
case NORTH:
|
|
getHandle().setDirection(EnumDirection.NORTH);
|
|
break;
|
|
case EAST:
|
|
getHandle().setDirection(EnumDirection.EAST);
|
|
break;
|
|
default:
|
|
throw new IllegalArgumentException(String.format("%s is not a valid facing direction", face));
|
|
}
|
|
if (!force && !getHandle().generation && !hanging.survives()) {
|
|
// Revert since it doesn't fit
|
|
hanging.setDirection(dir);
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
|
|
@Override
|
|
public BlockFace getFacing() {
|
|
EnumDirection direction = this.getHandle().getDirection();
|
|
if (direction == null) return BlockFace.SELF;
|
|
return CraftBlock.notchToBlockFace(direction);
|
|
}
|
|
|
|
@Override
|
|
public EntityHanging getHandle() {
|
|
return (EntityHanging) entity;
|
|
}
|
|
|
|
@Override
|
|
public String toString() {
|
|
return "CraftHanging";
|
|
}
|
|
|
|
@Override
|
|
public EntityType getType() {
|
|
return EntityType.UNKNOWN;
|
|
}
|
|
}
|