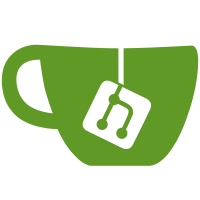
See the corresponding Bukkit commit for details. Implementation details: - Any packets that include an itemstack will send air stacks as null; maybe this will even eliminate the client crash that occurs if the client receives an air stack - Better handling of null itemstacks in general (ie less converting them to air stacks) - Inventory.setContents() can now take an array smaller than the inventory without error - Player.updateInventory() should now correctly update the result slot in a crafting inventory Some small credit goes to Afforess (initial implementation of openInventory() methods) and Drakia (initial implementation of InventoryOpenEvent and InventoryCloseEvent).
126 lines
3.7 KiB
Java
126 lines
3.7 KiB
Java
package net.minecraft.server;
|
|
|
|
// CraftBukkit start
|
|
import org.bukkit.craftbukkit.inventory.CraftInventoryFurnace;
|
|
import org.bukkit.craftbukkit.inventory.CraftInventoryView;
|
|
// CraftBukkit end
|
|
|
|
public class ContainerFurnace extends Container {
|
|
|
|
public TileEntityFurnace a; // CraftBukkit - Private -> Public
|
|
private int b = 0;
|
|
private int c = 0;
|
|
private int h = 0;
|
|
// CraftBukkit start
|
|
private CraftInventoryView bukkitEntity = null;
|
|
private PlayerInventory player;
|
|
// CraftBukkit end
|
|
|
|
public ContainerFurnace(PlayerInventory playerinventory, TileEntityFurnace tileentityfurnace) {
|
|
this.a = tileentityfurnace;
|
|
this.a(new Slot(tileentityfurnace, 0, 56, 17));
|
|
this.a(new Slot(tileentityfurnace, 1, 56, 53));
|
|
this.a(new SlotResult2(playerinventory.d, tileentityfurnace, 2, 116, 35));
|
|
this.player = playerinventory; // CraftBukkit - save player
|
|
|
|
int i;
|
|
|
|
for (i = 0; i < 3; ++i) {
|
|
for (int j = 0; j < 9; ++j) {
|
|
this.a(new Slot(playerinventory, j + i * 9 + 9, 8 + j * 18, 84 + i * 18));
|
|
}
|
|
}
|
|
|
|
for (i = 0; i < 9; ++i) {
|
|
this.a(new Slot(playerinventory, i, 8 + i * 18, 142));
|
|
}
|
|
}
|
|
|
|
public void a(ICrafting icrafting) {
|
|
super.a(icrafting);
|
|
icrafting.a(this, 0, this.a.cookTime);
|
|
icrafting.a(this, 1, this.a.burnTime);
|
|
icrafting.a(this, 2, this.a.ticksForCurrentFuel);
|
|
}
|
|
|
|
public void a() {
|
|
super.a();
|
|
|
|
for (int i = 0; i < this.listeners.size(); ++i) {
|
|
ICrafting icrafting = (ICrafting) this.listeners.get(i);
|
|
|
|
if (this.b != this.a.cookTime) {
|
|
icrafting.a(this, 0, this.a.cookTime);
|
|
}
|
|
|
|
if (this.c != this.a.burnTime) {
|
|
icrafting.a(this, 1, this.a.burnTime);
|
|
}
|
|
|
|
if (this.h != this.a.ticksForCurrentFuel) {
|
|
icrafting.a(this, 2, this.a.ticksForCurrentFuel);
|
|
}
|
|
}
|
|
|
|
this.b = this.a.cookTime;
|
|
this.c = this.a.burnTime;
|
|
this.h = this.a.ticksForCurrentFuel;
|
|
}
|
|
|
|
public boolean b(EntityHuman entityhuman) {
|
|
if (!this.checkReachable) return true; // CraftBukkit
|
|
return this.a.a(entityhuman);
|
|
}
|
|
|
|
public ItemStack a(int i) {
|
|
ItemStack itemstack = null;
|
|
Slot slot = (Slot) this.e.get(i);
|
|
|
|
if (slot != null && slot.c()) {
|
|
ItemStack itemstack1 = slot.getItem();
|
|
|
|
itemstack = itemstack1.cloneItemStack();
|
|
if (i == 2) {
|
|
if (!this.a(itemstack1, 3, 39, true)) {
|
|
return null;
|
|
}
|
|
} else if (i >= 3 && i < 30) {
|
|
if (!this.a(itemstack1, 30, 39, false)) {
|
|
return null;
|
|
}
|
|
} else if (i >= 30 && i < 39) {
|
|
if (!this.a(itemstack1, 3, 30, false)) {
|
|
return null;
|
|
}
|
|
} else if (!this.a(itemstack1, 3, 39, false)) {
|
|
return null;
|
|
}
|
|
|
|
if (itemstack1.count == 0) {
|
|
slot.c((ItemStack) null);
|
|
} else {
|
|
slot.d();
|
|
}
|
|
|
|
if (itemstack1.count == itemstack.count) {
|
|
return null;
|
|
}
|
|
|
|
slot.b(itemstack1);
|
|
}
|
|
|
|
return itemstack;
|
|
}
|
|
|
|
// CraftBukkit start
|
|
public CraftInventoryView getBukkitView() {
|
|
if (bukkitEntity != null) {
|
|
return bukkitEntity;
|
|
}
|
|
CraftInventoryFurnace inventory = new CraftInventoryFurnace(this.a);
|
|
bukkitEntity = new CraftInventoryView(this.player.d.getBukkitEntity(), inventory, this);
|
|
return bukkitEntity;
|
|
}
|
|
// CraftBukkit end
|
|
}
|